Introduction to Python Math for Data Science
Understanding fundamental math concepts is crucial for mastering data science, and Python provides an extensive toolkit to simplify mathematical operations. This blog will guide you through the Essential Python Math Concepts for Data Science, helping you leverage Python’s mathematical capabilities effectively.
Table of Contents
- Introduction to Python Math for Data Science
- Basic Arithmetic Operations in Python
- Advanced Mathematical Functions Using the
math
Module - Linear Algebra with NumPy
- Statistics and Probability in Python
- External Resources and Tools for Python Math
- Bonus Tips for Efficient Math Operations in Python
- Conclusion
Python is a popular programming language in data science due to its simplicity and robust libraries. Math plays a vital role in data manipulation, analysis, and machine learning, making Python’s mathematical capabilities indispensable. In this blog, we will explore key Python math concepts essential for data science tasks.
Basic Arithmetic Operations in Python
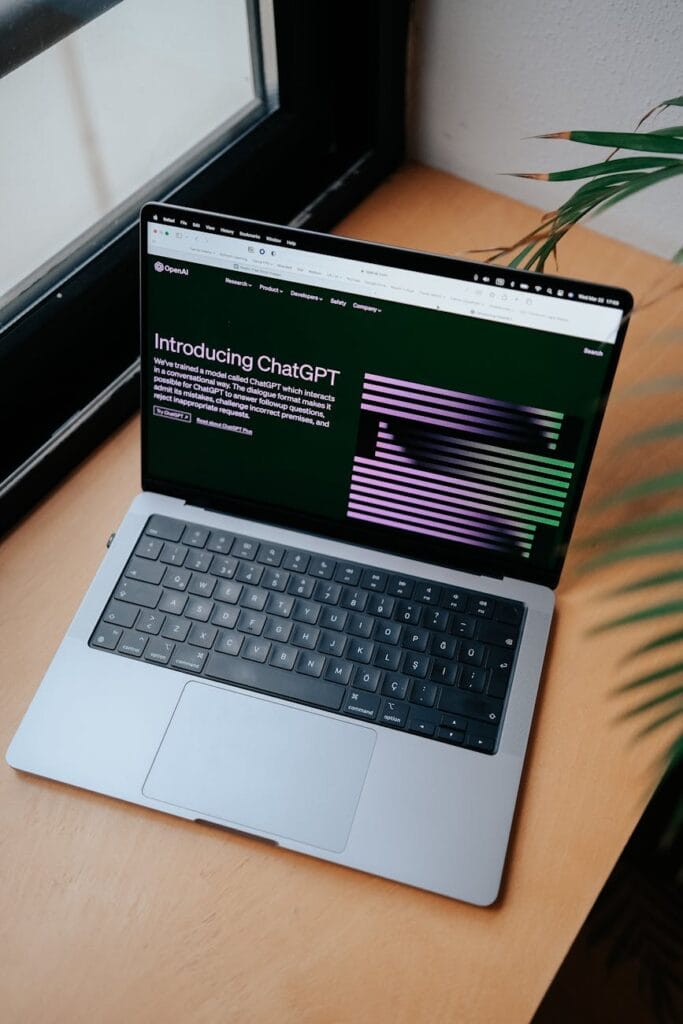
Basic Arithmetic Operations
Python supports basic arithmetic operations like addition, subtraction, multiplication, and division:
# Basic operations
x = 15
y = 4
print("Addition:", x + y) # Output: 19
print("Subtraction:", x - y) # Output: 11
print("Multiplication:", x * y) # Output: 60
print("Division:", x / y) # Output: 3.75
Key Operations
- Exponentiation:
**
(e.g.,3**2
gives 9) - Floor Division:
//
(e.g.,15//4
gives 3) - Modulo:
%
(remainder of the division, e.g.,15%4
gives 3)
Tip: Use parentheses to control the order of operations and make your code easier to read. For example, 3 + 4 * 2
computes differently than (3 + 4) * 2
.
Advanced Mathematical Functions Using the math
Module
The math
module in Python offers advanced mathematical operations:
Common Functions
- Square Root:
math.sqrt(x)
- Logarithms:
math.log(x)
(natural log),math.log10(x)
(base-10 log) - Trigonometric Functions:
math.sin(x)
,math.cos(x)
,math.tan(x)
Example:
import math
# Square root and log
num = 16
print("Square Root:", math.sqrt(num)) # Output: 4.0
print("Natural Log:", math.log(num)) # Output: 2.772588722239781
Tip: Use math.isclose()
for comparing floating-point numbers to avoid errors due to precision issues.
Linear Algebra with NumPy
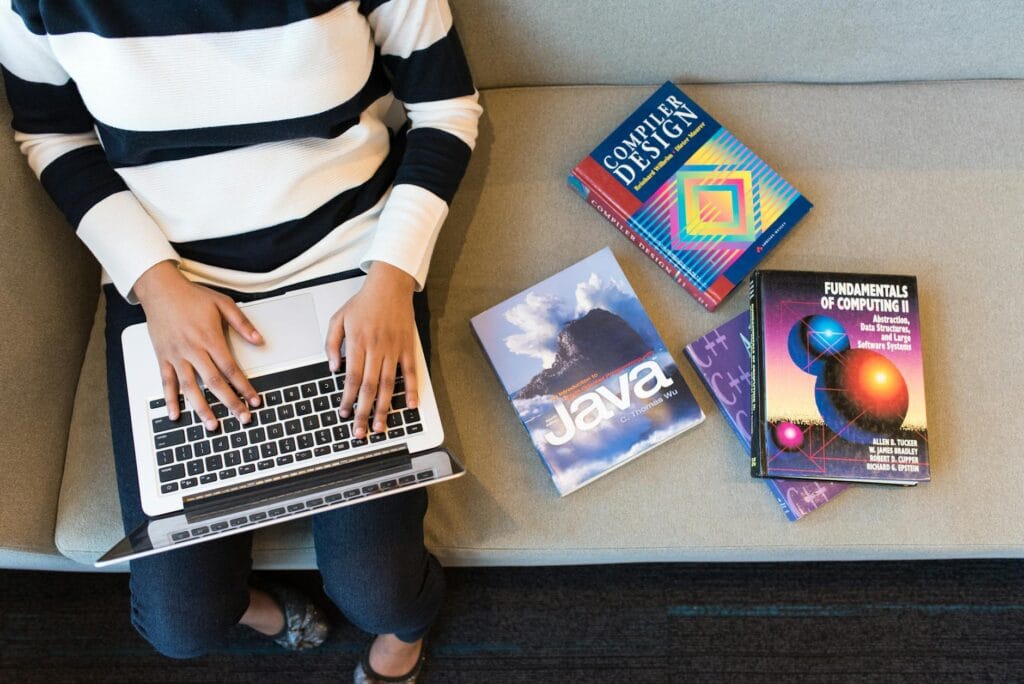
Linear Algebra
Linear algebra is a cornerstone of data science, particularly in machine learning. The NumPy
library simplifies matrix operations, vector calculations, and more.
Key Features
- Matrix Multiplication:
numpy.dot()
- Determinants:
numpy.linalg.det()
- Eigenvalues and Eigenvectors:
numpy.linalg.eig()
Example:
import numpy as np
# Matrix multiplication
A = np.array([[1, 2], [3, 4]])
B = np.array([[5, 6], [7, 8]])
print("Matrix Multiplication:\n", np.dot(A, B))
Tip: When working with large datasets, use sparse matrices from the scipy.sparse
module to save memory and improve performance.
Statistics and Probability in Python
Python excels in statistical analysis, thanks to libraries like NumPy
, Pandas
, and SciPy
.
Descriptive Statistics
- Mean:
numpy.mean(data)
- Median:
numpy.median(data)
- Standard Deviation:
numpy.std(data)
Probability Distributions
The SciPy
library offers functions for probability distributions:
- Normal Distribution:
scipy.stats.norm
- Binomial Distribution:
scipy.stats.binom
Example:
from scipy import stats
# Normal Distribution
mean = 0
std_dev = 1
print("CDF:", stats.norm.cdf(1.96, loc=mean, scale=std_dev))
Tip: Use pandas.DataFrame.describe()
for a quick summary of your data, including count, mean, standard deviation, and more.
External Resources and Tools for Python Math
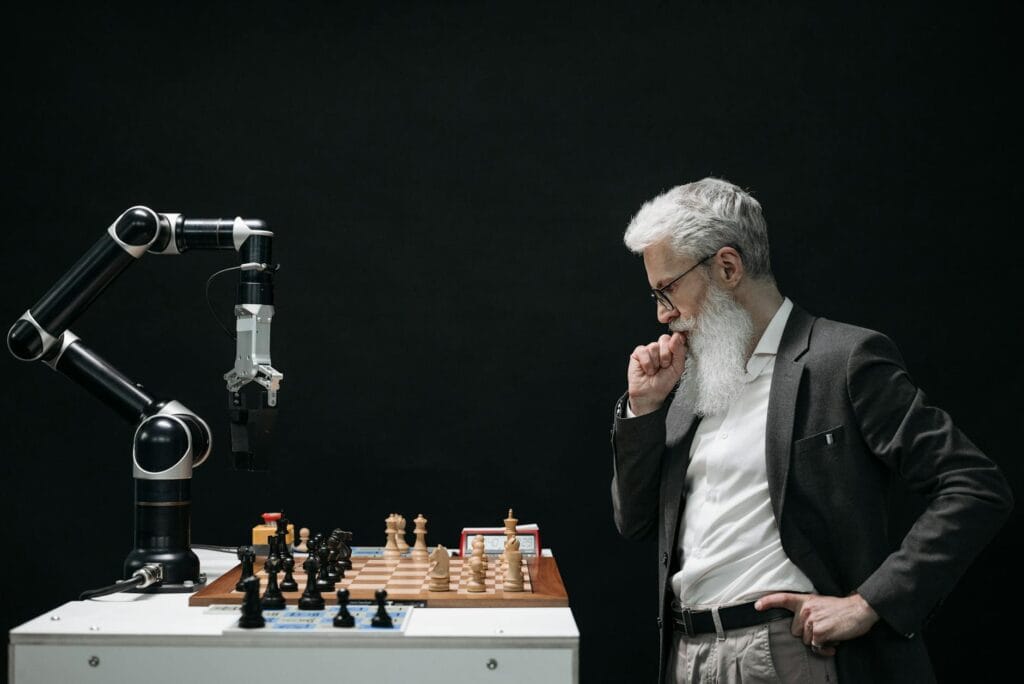
External Resources and Tools
Libraries to Explore
- SymPy: For symbolic mathematics.
- Matplotlib: For visualizing mathematical functions.
- Pandas: For data manipulation with statistical summaries.
External References
Tip: Explore online courses and tutorials to deepen your understanding of mathematical concepts and their applications in Python.
Bonus Tips for Efficient Math Operations in Python
- Use Vectorization: Replace loops with vectorized operations using NumPy for faster computation.
- Leverage Broadcasting: Simplify operations on arrays of different shapes with NumPy broadcasting.
- Optimize with Libraries: Use specialized libraries like
Numba
orCython
to accelerate numerical computations. - Profile Your Code: Use tools
cProfile
to identify bottlenecks in your mathematical computations.
Conclusion
Mastering math concepts in Python is a fundamental skill for data science professionals. From basic arithmetic to advanced linear algebra and statistics, Python provides the tools necessary to tackle complex data challenges. By leveraging libraries like NumPy and SciPy, you can efficiently analyze data and implement machine learning algorithms.
Harness the power of Python Math Concepts for Data Science to take your data science projects to the next level!
Your article helped me a lot, is there any more related content? Thanks!
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.