Introduction
Writing clean code in Python is not just a best practice; it’s an essential habit for creating reliable, maintainable, and scalable software. Clean code ensures clarity, reduces debugging time, and facilitates collaboration among developers. This guide explores the top practices for writing clean Python code and highlights common pitfalls to avoid.
Table of Contents
- Why Clean Code Matters
- Top Practices for Clean Code in Python
- Use Descriptive and Meaningful Names
- Adhere to the PEP 8 Style Guide
- Keep Functions Focused and Modular
- Utilize Python’s Built-in Libraries
- Avoidable Pitfalls in Python Coding
- Lack of Error Handling
- Overusing Global Variables
- Compromising Readability
- Conclusion
Why Clean Code Matters
Clean code is the foundation of high-quality software development. It offers several advantages:
- Improved Readability: Clean code is easier to understand, even for developers unfamiliar with the project.
- Simplified Maintenance: It reduces technical debt, making updating or extending functionality easier.
- Enhanced Debugging: Clear and organized code simplifies identifying and fixing issues.
Whether you are an individual contributor or part of a team, clean coding practices will set you apart as a proficient developer.
Top Practices for Clean Code in Python
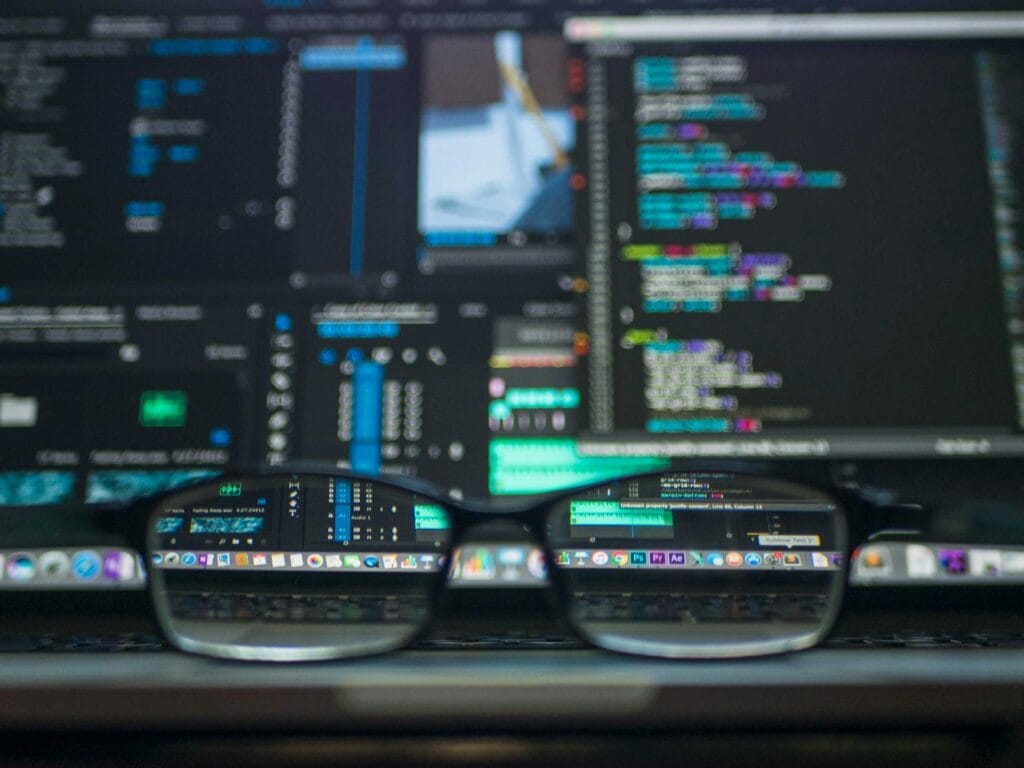
Clean Code in Python
Use Descriptive and Meaningful Names
Variable, function, and class names should clearly convey their purpose. Avoid vague or overly short names that can confuse other developers.
Example:
# Poor Practice
def calc(a, b):
return a + b
# Better Practice
def calculate_total_price(price, tax):
return price + tax
Good naming conventions save time and reduce misunderstandings in collaborative environments.
Adhere to the PEP 8 Style Guide
Python’s PEP 8 style guide provides detailed recommendations for writing consistent and clean code. Key points include:
- Use 4 spaces for indentation.
- Limit line length to 79 characters.
- Use blank lines to separate logical sections.
- Follow naming conventions (e.g.,
snake_case
for variables and functions,CamelCase
for classes).
External Resource: PEP 8 Style Guide
Keep Functions Focused and Modular
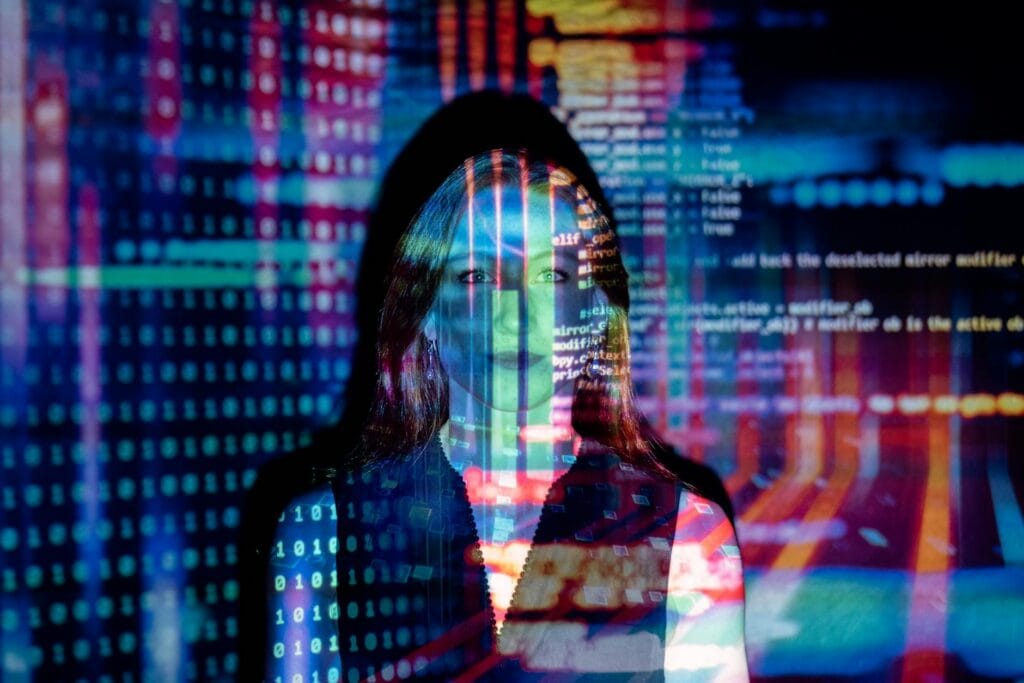
Focused and Modular
Each function should serve a single purpose. Avoid cramming multiple responsibilities into one function, as it can make the code harder to test and maintain.
Example:
# Poor Practice
def process_order(order):
validate_order(order)
save_to_database(order)
send_notification(order)
# Better Practice
def validate_order(order):
# Validation logic
def save_to_database(order):
# Database logic
def send_notification(order):
# Notification logic
Breaking down functions into smaller units makes your code more reusable and easier to test.
Utilize Python’s Built-in Libraries
Before reinventing the wheel, explore Python’s extensive standard library. Built-in modules often provide reliable and optimized solutions.
Example:
import os
# Use os for file management
files = os.listdir("/path/to/directory")
Leveraging these libraries not only saves development time but also improves code quality.
Avoidable Pitfalls in Python Coding
Lack of Error Handling
Failing to account for exceptions can lead to unpredictable behavior or crashes. Proper error handling ensures your program behaves gracefully under unexpected conditions.
Example:
# Poor Practice
number = int(input("Enter a number: ")) / 0
# Better Practice
try:
number = int(input("Enter a number: ")) / 0
except ZeroDivisionError:
print("Error: Division by zero is not allowed.")
except ValueError:
print("Error: Invalid input. Please enter a number.")
Overusing Global Variables
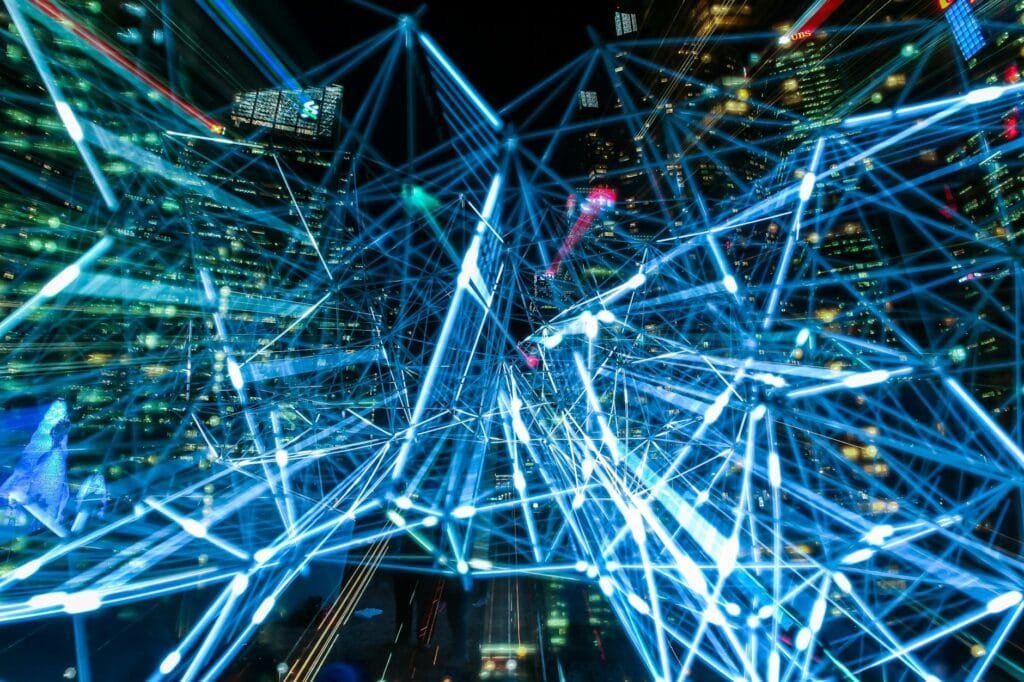
Global Variables
Global variables can make your code difficult to debug and maintain. Instead, use local variables and pass data explicitly between functions.
Example:
# Poor Practice
x = 5
def increment():
global x
x += 1
# Better Practice
def increment(value):
return value + 1
Reducing dependency on the global state makes your code more predictable and easier to test.
Compromising Readability
Avoid overly complex expressions or nested logic that can confuse readers. Aim for simplicity and clarity in every line of code.
Example:
# Poor Practice
result = [x**2 for x in range(20) if x % 2 == 0]
# Better Practice
result = []
for x in range(20):
if x % 2 == 0:
result.append(x**2)
Readable code ensures that anyone revisiting your work can understand it without extensive context.
Conclusion
Clean code is a critical skill that every Python developer should cultivate. By following the practices discussed in this guide—such as using meaningful names, adhering to PEP 8, writing modular functions, and avoiding common pitfalls—you can create efficient, maintainable, and professional code.
Start applying these principles to your projects today. The effort you invest in writing clean code will pay off in the long run, enhancing your productivity and reputation as a developer.
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?