Welcome, coders and tech enthusiasts! If you’re looking to supercharge your programming skills, you’re in the right place. In this post, we’ll explore 18 programming concepts you may not have heard of but should get familiar with. Each concept will expand your understanding of code and prepare you to solve complex problems more effectively.
Table of Contents
- Null Object Pattern
- Continuation-Passing Style
- Aspect-Oriented Programming
- Code Contracts
- Functors
- Monads
- Idempotence
- Memoization
- Combinator Patterns
- Prototype Pattern
- Lazy Evaluation
- Dependency Injection
- Domain-Specific Languages
- Event Sourcing
- Backpropagation
- Genetic Algorithms
- Probabilistic Programming
- Reactive Programming
1. Null Object Pattern
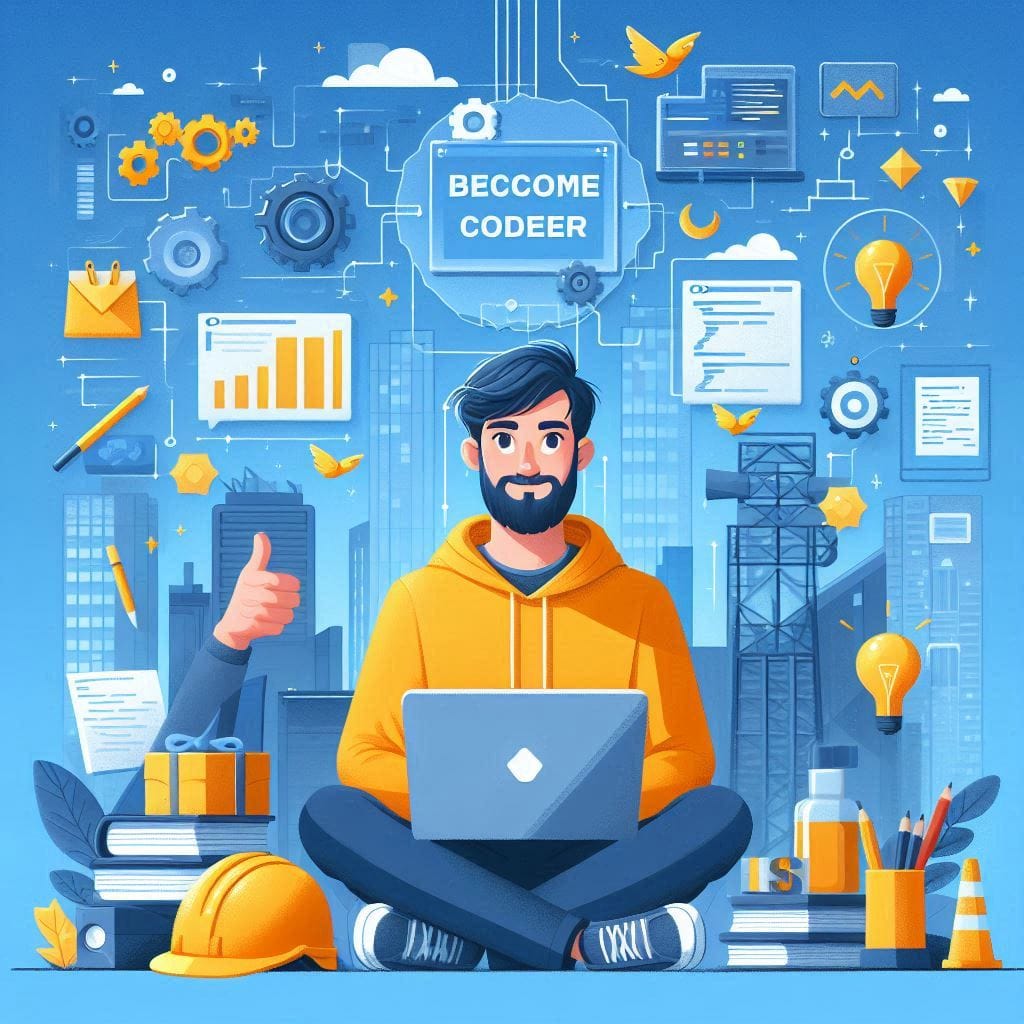
Null Object Pattern
The Null Object Pattern simplifies your code by providing an alternative object for “null” references, helping avoid null pointer exceptions. This pattern is useful in languages where null values can lead to unexpected bugs. With a null object, you can eliminate many conditional checks.
2. Continuation-Passing Style (CPS)
In the Continuation-Passing Style, functions don’t return results directly but pass them to a continuation function. CPS can simplify asynchronous operations widely used in functional programming, especially in languages like JavaScript where callbacks and promises are common.
3. Aspect-Oriented Programming (AOP)
Aspect-oriented programming allows for the separation of cross-cutting concerns such as logging, security, and transaction management, making your codebase cleaner and more modular. Frameworks like Spring AOP support this paradigm.
4. Code Contracts
Using code contracts, developers define conditions for method inputs, outputs, and invariants. Tools like Microsoft’s Code Contracts library can help enforce these rules at runtime, improving code reliability and robustness.
5. Functors
A functor is an object that can be mapped over, applying a function to values within a context. Functors are foundational in functional programming languages like Haskell and Scala, where they help to transform data structures without altering the structure itself.
6. Monads
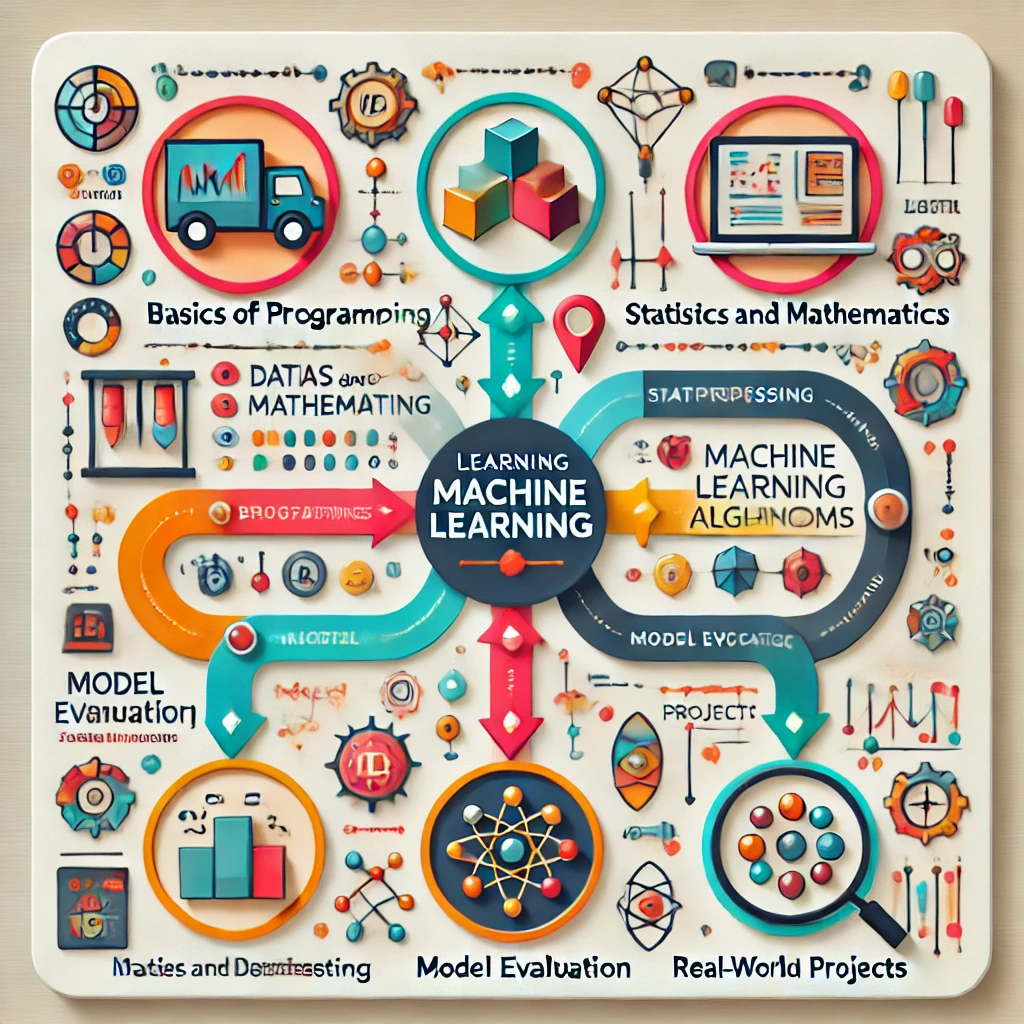
Monads
A monad is a design pattern that helps handle side effects in functional programming by wrapping values and chaining operations. Monads make it easier to work with operations that may fail, return nothing, or involve asynchronous actions.
7. Idempotence
In programming, idempotence refers to operating multiple times without changing the outcome. This property is crucial in network communications and database operations to prevent unintended state changes from repeated requests.
8. Memoization
Memoization is a caching technique to store the results of expensive function calls and return them when the same inputs occur again. This concept improves performance, especially in recursive functions where repeated calculations are common.
9. Combinator Patterns
Combinator patterns involve combining simpler functions to build more complex ones. In functional programming, combinators can reduce redundancy and make code modular and reusable. Examples include map, reduce, and filter functions.
10. Prototype Pattern
The Prototype Pattern is a creational pattern that uses cloning to create new objects based on an existing instance. Common in JavaScript, this pattern helps when you want multiple instances that share similar structure but have individual attributes.
11. Lazy Evaluation
Lazy evaluation delays the computation of expressions until their values are needed. This can lead to performance improvements by avoiding unnecessary calculations, especially in languages like Python and Haskell where lazy evaluation is standard.
12. Dependency Injection (DI)

Dependency Injection (DI)
Dependency Injection is a design pattern where dependencies are injected into a class from the outside rather than being hard-coded. DI fosters modularity and makes unit testing easier. Many frameworks, like Angular and Spring, use DI to manage dependencies.
13. Domain-Specific Languages (DSLs)
A Domain-Specific Language is a small language specialized to a specific domain. Examples include SQL for databases and HTML for web pages. DSLs allow developers to express their intent more directly and understandably in certain contexts.
14. Event Sourcing
In Event Sourcing, the application state is stored as a sequence of events rather than a single state snapshot. This approach makes it easy to audit and replay events to recreate previous states, popular in applications with strict audit requirements.
15. Backpropagation
While often associated with machine learning, backpropagation is the algorithm that allows neural networks to adjust weights based on the error rate of predictions. It’s foundational to training deep learning models.
16. Genetic Algorithms
Genetic Algorithms are inspired by the process of natural selection. They’re used to solve optimization and search problems by evolving solutions over time, often applied in fields like artificial intelligence and operations research.
17. Probabilistic Programming
Probabilistic Programming allows you to work with uncertainty by modeling problems with probabilistic variables. Languages like PyMC3 and Stan are specialized for probabilistic programming, and the approach is valuable in machine learning and data science.
18. Reactive Programming
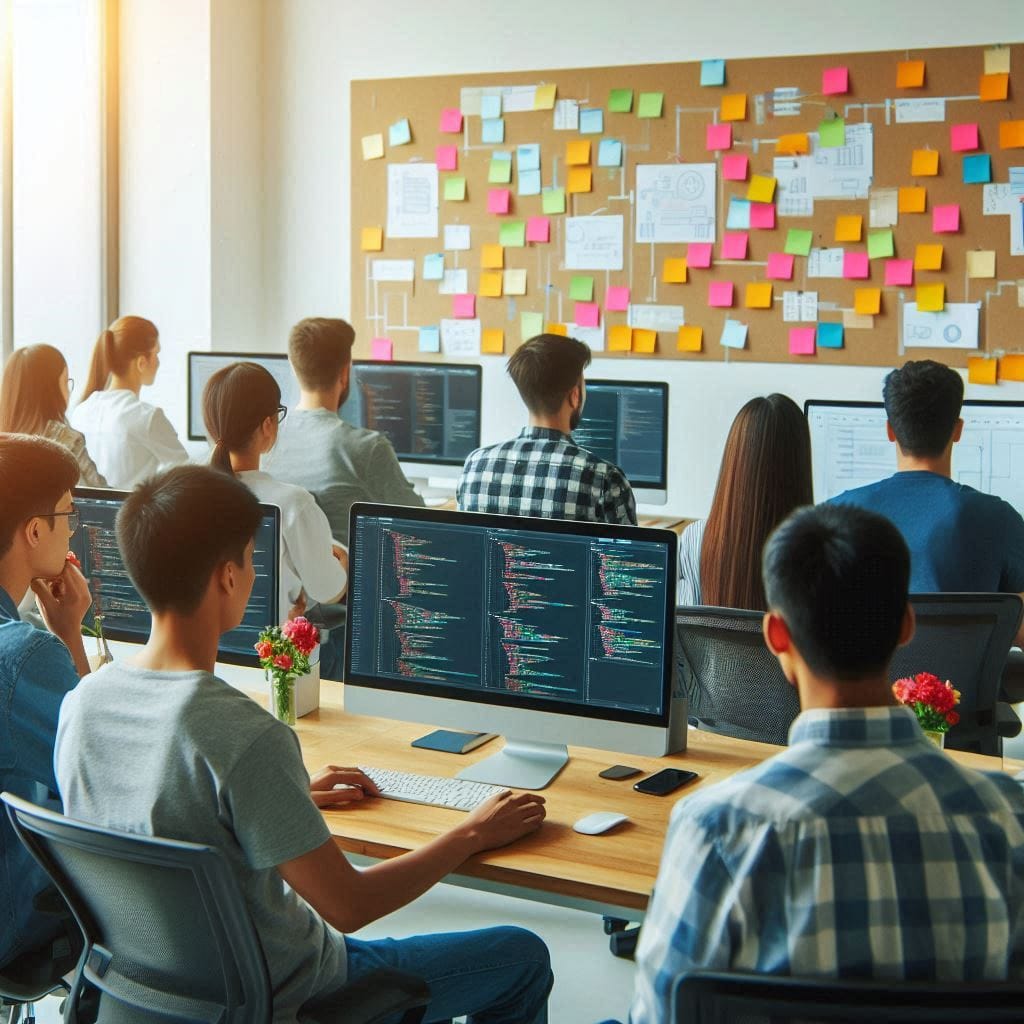
Reactive Programming
Reactive Programming is an asynchronous programming paradigm centered on data streams and the propagation of change. Libraries like RxJS (JavaScript) and ReactiveX enable developers to work with streams and handle asynchronous data flows more intuitively.
Why These Concepts Matter
Understanding these programming concepts can significantly enhance your problem-solving skills. With concepts like null object patterns and memoization, you’ll be able to build more efficient, bug-free applications. Others, like monads and continuation-passing style, allow you to think differently about data manipulation, especially in complex, functional programming scenarios.
By learning about concepts like probabilistic programming and reactive programming, you can also prepare for advancements in fields like data science, artificial intelligence, and real-time data processing. Concepts like backpropagation are particularly crucial for developers in AI, as they underpin machine learning algorithms used today.
Additional Resources
- Introduction to Functional Programming – Understand more about functional programming concepts like monads and functors.
- Domain-Specific Language Overview – Learn why DSLs can simplify complex domains.
- Understanding Reactive Programming – Explore how reactive programming frameworks work in various languages.
With these resources, you can dive deeper into each concept and see examples of their applications in real-world scenarios.
Internal Resources
If you’re interested in exploring more, check out our posts on:
Conclusion
Programming is an ever-evolving field, and mastering these 18 lesser-known concepts could give you a competitive edge. By exploring these concepts, you not only expand your knowledge but also learn to solve problems in innovative ways. Bookmark this guide, and revisit these concepts whenever you need inspiration or a fresh perspective on a programming problem.
Your article helped me a lot, is there any more related content? Thanks!
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
yes, ofcourse
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.